Quick Tip: Text Generation with Rant (C#) in Python
Recently, on Hacker News, I stumbled across Rant, which is a very interesting procedural text generation engine written in C#. While I have no actual use-case at the moment, it is still seemed like a great toy to play with (for now)!
Rant is both a C# library (including some tools) as well as a fairly advanced and feature-rich query language.
At its core, Rant helps you with all sorts of string (text) generation tasks. Aside from more fundamental features such as capitalization, Rant also can handle tasks such as (random) dialogue generation, rhyming, and automatic formatting.
One of the coolest, albeit very simple, features of Rant is its ability to query so-called dictionaries. You could, for example, ask Rant for a <noun-animal>
and it would check one of its (customizable) dictionaries for a fitting lexeme or construction. This is implemented as a very complex and powerful slot-filler model.
All of that being said, this article is not about Rant’s features. Since most of my coding happens in Python, I wanted to avoid a journey into C#. Hence this short tutorial will be about using Rant in Python.
How to Use Rant in Python
Please be aware that the following tutorial is targeted towards Windows! I am reasonably certain, however, that there is a Mono solution as well!
While running C code in Python is no big deal, C# poses some challenges. Luckily, there is a library called pythonnet
which gives us an almost seamless integration with .NET’s Common Language Runtime. This library can, thanks to some Python magic, be installed via pip
without any trouble.
Aside from this library, you will also need a Rant.dll
and a Rantionary package. While Rant supports highly customized dictionaries, Rationary is the default one that ships with the library.
Unfortunately, the precompiled versions of both of these seem to be outdated. Hence, we are going to compile Rant ourselves.
In order to do this, you will need to have Visual Studio (Community Edition) installed on your computer.
Will will use Git to clone both Rant and Rantionary right from GitHub:
git clone https://github.com/TheBerkin/rant.git
git clone https://github.com/RantLang/Rantionary.git
Now, open the Rant project (Rant.sln
) in VS and build both Rant and the Rant Tools, which will be needed to package the dictionary.
In the bin/Release
folder you should now find both the Rant.dll
as well as the command line tool RCT.exe
. This tool can be used to package the previously cloned Rantionary dictionary.
Open a command prompt and run:
rct.exe pack Rantionary
This will create a file (package) called Rantionary-X.X.X.rantpkg
. Now that we have all the parts needed, we can look at a Python example:
import clr
clr.AddReference('Rant')
from Rant import RantEngine, RantProgram
rant = RantEngine()
rant.LoadPackage('Rantionary-3.1.0.rantpkg')
rant.Dictionary.IncludeHiddenClass('nsfw')
def run_rant_pattern(pattern):
rp = RantProgram.CompileString(pattern)
return rant.Do(rp)
if __name__ == '__main__':
simple_pattern = run_rant_pattern('<name-female> really likes Python!')
semi_complex_pattern = run_rant_pattern('<name-female> <verb.ed-transitive> <name> with <pro.dposs-female> <adj> <noun>.')
print(simple_pattern)
print(semi_complex_pattern)
First, we are using pythonnet
(clr
) to load Rant.dll
. From now on, we can treat Rant as if it were a regular Python library. Hence, we can simply import both RantEngine
and RantProgram
.
Next, we are instantiating a RantEngine object and also load the default dictionary (with ‘nsfw’ words enabled).
Rant-patterns (essentially strings) need to be compiled before they can be “run”. Hence, I wrote, for demonstration purposes, a little helper function called run_rant_pattern
.
Finally, we are running and printing two Rant-patterns. The first one is very straight forward and just creates a simple sentence with a random female name as the subject (e.g. “Mary really likes Python!”).
In the second example, we are generating a more complex sentencewith multiple (random) entities and a transitive verb (= requires an object) in the past tense.
For your convenience and enjoyment, here are ten examples of the beautiful prose this simple pattern generated:
- Dakota strapped Tiffanie with her keen hotel.
- Daijah vaporized Odalys with her large microwave.
- Latricia swiped Claudio with their jelly-belly roof.
- Gustie drafted Araminta with her irregular bathroom.
- Petra tapped Arland with her gassy submarine.
- Nanie jet-sprayed Krystle with their legitimate boulder.
- Pearlie stabbed Abb with their ethical broom.
- Baby annihilated Harry with their nasty frown.
- Nyla breastfed Leta with their corny blank face.
- Shanita cleaned Jess with her rough cockroach.
Well, at least some of those could be considered … artsy?
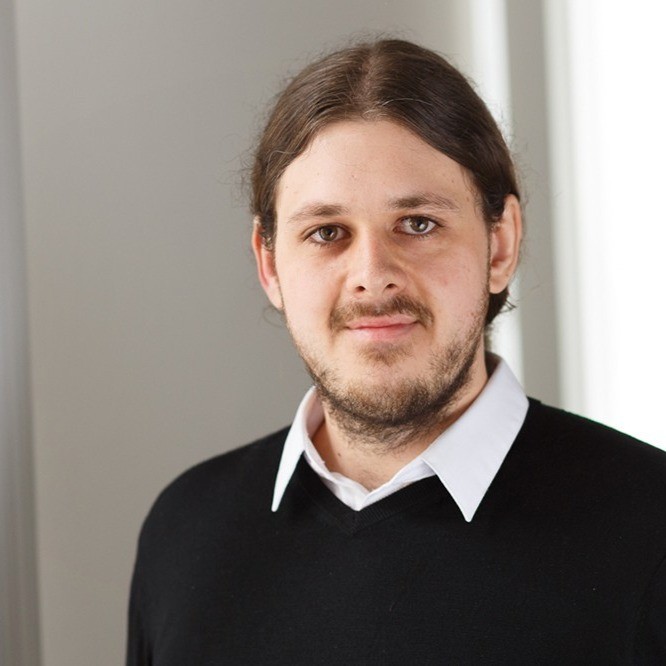
Thank you for visiting!
I hope, you are enjoying the article! I'd love to get in touch! 😀
Follow me on LinkedIn